2019-11-01 23:51:22 +01:00
|
|
|
// Copyright 2019 The Gitea Authors. All rights reserved.
|
2022-11-27 19:20:29 +01:00
|
|
|
// SPDX-License-Identifier: MIT
|
2019-11-01 23:51:22 +01:00
|
|
|
|
|
|
|
package webhook
|
|
|
|
|
|
|
|
import (
|
2022-05-20 16:08:52 +02:00
|
|
|
"context"
|
2023-07-28 19:46:48 +02:00
|
|
|
"errors"
|
2019-11-01 23:51:22 +01:00
|
|
|
"fmt"
|
2024-03-07 23:18:38 +01:00
|
|
|
"net/http"
|
2019-11-01 23:51:22 +01:00
|
|
|
"strings"
|
|
|
|
|
2023-11-24 04:49:41 +01:00
|
|
|
"code.gitea.io/gitea/models/db"
|
2021-12-10 02:27:50 +01:00
|
|
|
repo_model "code.gitea.io/gitea/models/repo"
|
2022-10-21 18:21:56 +02:00
|
|
|
user_model "code.gitea.io/gitea/models/user"
|
2021-11-10 06:13:16 +01:00
|
|
|
webhook_model "code.gitea.io/gitea/models/webhook"
|
2019-11-01 23:51:22 +01:00
|
|
|
"code.gitea.io/gitea/modules/git"
|
2022-04-25 20:03:01 +02:00
|
|
|
"code.gitea.io/gitea/modules/graceful"
|
2019-11-01 23:51:22 +01:00
|
|
|
"code.gitea.io/gitea/modules/log"
|
2024-03-02 16:42:31 +01:00
|
|
|
"code.gitea.io/gitea/modules/optional"
|
2022-04-25 20:03:01 +02:00
|
|
|
"code.gitea.io/gitea/modules/queue"
|
2019-11-01 23:51:22 +01:00
|
|
|
"code.gitea.io/gitea/modules/setting"
|
|
|
|
api "code.gitea.io/gitea/modules/structs"
|
2021-08-12 14:43:08 +02:00
|
|
|
"code.gitea.io/gitea/modules/util"
|
2023-01-01 16:23:15 +01:00
|
|
|
webhook_module "code.gitea.io/gitea/modules/webhook"
|
2021-08-12 14:43:08 +02:00
|
|
|
|
2019-11-01 23:51:22 +01:00
|
|
|
"github.com/gobwas/glob"
|
|
|
|
)
|
|
|
|
|
2024-03-07 23:18:38 +01:00
|
|
|
var webhookRequesters = map[webhook_module.HookType]func(context.Context, *webhook_model.Webhook, *webhook_model.HookTask) (req *http.Request, body []byte, err error){
|
|
|
|
webhook_module.SLACK: newSlackRequest,
|
|
|
|
webhook_module.DISCORD: newDiscordRequest,
|
|
|
|
webhook_module.DINGTALK: newDingtalkRequest,
|
|
|
|
webhook_module.TELEGRAM: newTelegramRequest,
|
|
|
|
webhook_module.MSTEAMS: newMSTeamsRequest,
|
|
|
|
webhook_module.FEISHU: newFeishuRequest,
|
|
|
|
webhook_module.MATRIX: newMatrixRequest,
|
|
|
|
webhook_module.WECHATWORK: newWechatworkRequest,
|
|
|
|
webhook_module.PACKAGIST: newPackagistRequest,
|
2022-01-20 18:46:10 +01:00
|
|
|
}
|
2020-12-08 11:41:14 +01:00
|
|
|
|
|
|
|
// IsValidHookTaskType returns true if a webhook registered
|
|
|
|
func IsValidHookTaskType(name string) bool {
|
2023-01-01 16:23:15 +01:00
|
|
|
if name == webhook_module.GITEA || name == webhook_module.GOGS {
|
2020-12-12 16:33:19 +01:00
|
|
|
return true
|
|
|
|
}
|
2024-03-07 23:18:38 +01:00
|
|
|
_, ok := webhookRequesters[name]
|
2020-12-08 11:41:14 +01:00
|
|
|
return ok
|
|
|
|
}
|
|
|
|
|
2019-11-02 03:35:12 +01:00
|
|
|
// hookQueue is a global queue of web hooks
|
Rewrite queue (#24505)
# ⚠️ Breaking
Many deprecated queue config options are removed (actually, they should
have been removed in 1.18/1.19).
If you see the fatal message when starting Gitea: "Please update your
app.ini to remove deprecated config options", please follow the error
messages to remove these options from your app.ini.
Example:
```
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].ISSUE_INDEXER_QUEUE_TYPE`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].UPDATE_BUFFER_LEN`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [F] Please update your app.ini to remove deprecated config options
```
Many options in `[queue]` are are dropped, including:
`WRAP_IF_NECESSARY`, `MAX_ATTEMPTS`, `TIMEOUT`, `WORKERS`,
`BLOCK_TIMEOUT`, `BOOST_TIMEOUT`, `BOOST_WORKERS`, they can be removed
from app.ini.
# The problem
The old queue package has some legacy problems:
* complexity: I doubt few people could tell how it works.
* maintainability: Too many channels and mutex/cond are mixed together,
too many different structs/interfaces depends each other.
* stability: due to the complexity & maintainability, sometimes there
are strange bugs and difficult to debug, and some code doesn't have test
(indeed some code is difficult to test because a lot of things are mixed
together).
* general applicability: although it is called "queue", its behavior is
not a well-known queue.
* scalability: it doesn't seem easy to make it work with a cluster
without breaking its behaviors.
It came from some very old code to "avoid breaking", however, its
technical debt is too heavy now. It's a good time to introduce a better
"queue" package.
# The new queue package
It keeps using old config and concept as much as possible.
* It only contains two major kinds of concepts:
* The "base queue": channel, levelqueue, redis
* They have the same abstraction, the same interface, and they are
tested by the same testing code.
* The "WokerPoolQueue", it uses the "base queue" to provide "worker
pool" function, calls the "handler" to process the data in the base
queue.
* The new code doesn't do "PushBack"
* Think about a queue with many workers, the "PushBack" can't guarantee
the order for re-queued unhandled items, so in new code it just does
"normal push"
* The new code doesn't do "pause/resume"
* The "pause/resume" was designed to handle some handler's failure: eg:
document indexer (elasticsearch) is down
* If a queue is paused for long time, either the producers blocks or the
new items are dropped.
* The new code doesn't do such "pause/resume" trick, it's not a common
queue's behavior and it doesn't help much.
* If there are unhandled items, the "push" function just blocks for a
few seconds and then re-queue them and retry.
* The new code doesn't do "worker booster"
* Gitea's queue's handlers are light functions, the cost is only the
go-routine, so it doesn't make sense to "boost" them.
* The new code only use "max worker number" to limit the concurrent
workers.
* The new "Push" never blocks forever
* Instead of creating more and more blocking goroutines, return an error
is more friendly to the server and to the end user.
There are more details in code comments: eg: the "Flush" problem, the
strange "code.index" hanging problem, the "immediate" queue problem.
Almost ready for review.
TODO:
* [x] add some necessary comments during review
* [x] add some more tests if necessary
* [x] update documents and config options
* [x] test max worker / active worker
* [x] re-run the CI tasks to see whether any test is flaky
* [x] improve the `handleOldLengthConfiguration` to provide more
friendly messages
* [x] fine tune default config values (eg: length?)
## Code coverage:
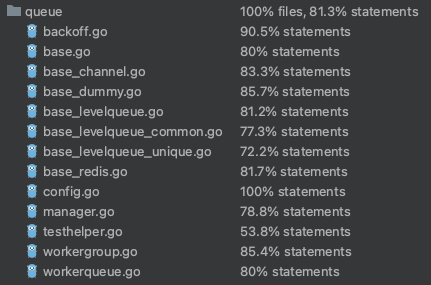
2023-05-08 13:49:59 +02:00
|
|
|
var hookQueue *queue.WorkerPoolQueue[int64]
|
2019-11-01 23:51:22 +01:00
|
|
|
|
|
|
|
// getPayloadBranch returns branch for hook event, if applicable.
|
|
|
|
func getPayloadBranch(p api.Payloader) string {
|
|
|
|
switch pp := p.(type) {
|
|
|
|
case *api.CreatePayload:
|
|
|
|
if pp.RefType == "branch" {
|
|
|
|
return pp.Ref
|
|
|
|
}
|
|
|
|
case *api.DeletePayload:
|
|
|
|
if pp.RefType == "branch" {
|
|
|
|
return pp.Ref
|
|
|
|
}
|
|
|
|
case *api.PushPayload:
|
|
|
|
if strings.HasPrefix(pp.Ref, git.BranchPrefix) {
|
|
|
|
return pp.Ref[len(git.BranchPrefix):]
|
|
|
|
}
|
|
|
|
}
|
|
|
|
return ""
|
|
|
|
}
|
|
|
|
|
2022-10-21 18:21:56 +02:00
|
|
|
// EventSource represents the source of a webhook action. Repository and/or Owner must be set.
|
|
|
|
type EventSource struct {
|
|
|
|
Repository *repo_model.Repository
|
|
|
|
Owner *user_model.User
|
|
|
|
}
|
2022-04-25 20:03:01 +02:00
|
|
|
|
2022-10-21 18:21:56 +02:00
|
|
|
// handle delivers hook tasks
|
Rewrite queue (#24505)
# ⚠️ Breaking
Many deprecated queue config options are removed (actually, they should
have been removed in 1.18/1.19).
If you see the fatal message when starting Gitea: "Please update your
app.ini to remove deprecated config options", please follow the error
messages to remove these options from your app.ini.
Example:
```
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].ISSUE_INDEXER_QUEUE_TYPE`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].UPDATE_BUFFER_LEN`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [F] Please update your app.ini to remove deprecated config options
```
Many options in `[queue]` are are dropped, including:
`WRAP_IF_NECESSARY`, `MAX_ATTEMPTS`, `TIMEOUT`, `WORKERS`,
`BLOCK_TIMEOUT`, `BOOST_TIMEOUT`, `BOOST_WORKERS`, they can be removed
from app.ini.
# The problem
The old queue package has some legacy problems:
* complexity: I doubt few people could tell how it works.
* maintainability: Too many channels and mutex/cond are mixed together,
too many different structs/interfaces depends each other.
* stability: due to the complexity & maintainability, sometimes there
are strange bugs and difficult to debug, and some code doesn't have test
(indeed some code is difficult to test because a lot of things are mixed
together).
* general applicability: although it is called "queue", its behavior is
not a well-known queue.
* scalability: it doesn't seem easy to make it work with a cluster
without breaking its behaviors.
It came from some very old code to "avoid breaking", however, its
technical debt is too heavy now. It's a good time to introduce a better
"queue" package.
# The new queue package
It keeps using old config and concept as much as possible.
* It only contains two major kinds of concepts:
* The "base queue": channel, levelqueue, redis
* They have the same abstraction, the same interface, and they are
tested by the same testing code.
* The "WokerPoolQueue", it uses the "base queue" to provide "worker
pool" function, calls the "handler" to process the data in the base
queue.
* The new code doesn't do "PushBack"
* Think about a queue with many workers, the "PushBack" can't guarantee
the order for re-queued unhandled items, so in new code it just does
"normal push"
* The new code doesn't do "pause/resume"
* The "pause/resume" was designed to handle some handler's failure: eg:
document indexer (elasticsearch) is down
* If a queue is paused for long time, either the producers blocks or the
new items are dropped.
* The new code doesn't do such "pause/resume" trick, it's not a common
queue's behavior and it doesn't help much.
* If there are unhandled items, the "push" function just blocks for a
few seconds and then re-queue them and retry.
* The new code doesn't do "worker booster"
* Gitea's queue's handlers are light functions, the cost is only the
go-routine, so it doesn't make sense to "boost" them.
* The new code only use "max worker number" to limit the concurrent
workers.
* The new "Push" never blocks forever
* Instead of creating more and more blocking goroutines, return an error
is more friendly to the server and to the end user.
There are more details in code comments: eg: the "Flush" problem, the
strange "code.index" hanging problem, the "immediate" queue problem.
Almost ready for review.
TODO:
* [x] add some necessary comments during review
* [x] add some more tests if necessary
* [x] update documents and config options
* [x] test max worker / active worker
* [x] re-run the CI tasks to see whether any test is flaky
* [x] improve the `handleOldLengthConfiguration` to provide more
friendly messages
* [x] fine tune default config values (eg: length?)
## Code coverage:
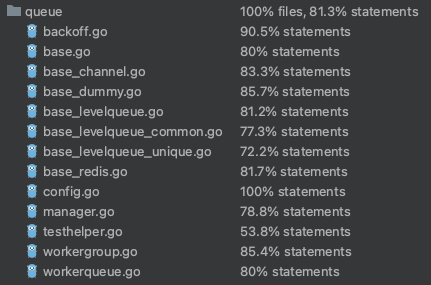
2023-05-08 13:49:59 +02:00
|
|
|
func handler(items ...int64) []int64 {
|
2022-10-21 18:21:56 +02:00
|
|
|
ctx := graceful.GetManager().HammerContext()
|
2022-04-25 20:03:01 +02:00
|
|
|
|
Rewrite queue (#24505)
# ⚠️ Breaking
Many deprecated queue config options are removed (actually, they should
have been removed in 1.18/1.19).
If you see the fatal message when starting Gitea: "Please update your
app.ini to remove deprecated config options", please follow the error
messages to remove these options from your app.ini.
Example:
```
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].ISSUE_INDEXER_QUEUE_TYPE`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [E] Removed queue option: `[indexer].UPDATE_BUFFER_LEN`. Use new options in `[queue.issue_indexer]`
2023/05/06 19:39:22 [F] Please update your app.ini to remove deprecated config options
```
Many options in `[queue]` are are dropped, including:
`WRAP_IF_NECESSARY`, `MAX_ATTEMPTS`, `TIMEOUT`, `WORKERS`,
`BLOCK_TIMEOUT`, `BOOST_TIMEOUT`, `BOOST_WORKERS`, they can be removed
from app.ini.
# The problem
The old queue package has some legacy problems:
* complexity: I doubt few people could tell how it works.
* maintainability: Too many channels and mutex/cond are mixed together,
too many different structs/interfaces depends each other.
* stability: due to the complexity & maintainability, sometimes there
are strange bugs and difficult to debug, and some code doesn't have test
(indeed some code is difficult to test because a lot of things are mixed
together).
* general applicability: although it is called "queue", its behavior is
not a well-known queue.
* scalability: it doesn't seem easy to make it work with a cluster
without breaking its behaviors.
It came from some very old code to "avoid breaking", however, its
technical debt is too heavy now. It's a good time to introduce a better
"queue" package.
# The new queue package
It keeps using old config and concept as much as possible.
* It only contains two major kinds of concepts:
* The "base queue": channel, levelqueue, redis
* They have the same abstraction, the same interface, and they are
tested by the same testing code.
* The "WokerPoolQueue", it uses the "base queue" to provide "worker
pool" function, calls the "handler" to process the data in the base
queue.
* The new code doesn't do "PushBack"
* Think about a queue with many workers, the "PushBack" can't guarantee
the order for re-queued unhandled items, so in new code it just does
"normal push"
* The new code doesn't do "pause/resume"
* The "pause/resume" was designed to handle some handler's failure: eg:
document indexer (elasticsearch) is down
* If a queue is paused for long time, either the producers blocks or the
new items are dropped.
* The new code doesn't do such "pause/resume" trick, it's not a common
queue's behavior and it doesn't help much.
* If there are unhandled items, the "push" function just blocks for a
few seconds and then re-queue them and retry.
* The new code doesn't do "worker booster"
* Gitea's queue's handlers are light functions, the cost is only the
go-routine, so it doesn't make sense to "boost" them.
* The new code only use "max worker number" to limit the concurrent
workers.
* The new "Push" never blocks forever
* Instead of creating more and more blocking goroutines, return an error
is more friendly to the server and to the end user.
There are more details in code comments: eg: the "Flush" problem, the
strange "code.index" hanging problem, the "immediate" queue problem.
Almost ready for review.
TODO:
* [x] add some necessary comments during review
* [x] add some more tests if necessary
* [x] update documents and config options
* [x] test max worker / active worker
* [x] re-run the CI tasks to see whether any test is flaky
* [x] improve the `handleOldLengthConfiguration` to provide more
friendly messages
* [x] fine tune default config values (eg: length?)
## Code coverage:
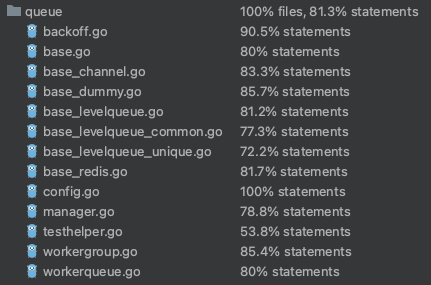
2023-05-08 13:49:59 +02:00
|
|
|
for _, taskID := range items {
|
|
|
|
task, err := webhook_model.GetHookTaskByID(ctx, taskID)
|
2022-04-25 20:03:01 +02:00
|
|
|
if err != nil {
|
2023-07-28 19:46:48 +02:00
|
|
|
if errors.Is(err, util.ErrNotExist) {
|
|
|
|
log.Warn("GetHookTaskByID[%d] warn: %v", taskID, err)
|
|
|
|
} else {
|
|
|
|
log.Error("GetHookTaskByID[%d] failed: %v", taskID, err)
|
|
|
|
}
|
2022-11-23 15:10:04 +01:00
|
|
|
continue
|
|
|
|
}
|
|
|
|
|
|
|
|
if task.IsDelivered {
|
|
|
|
// Already delivered in the meantime
|
|
|
|
log.Trace("Task[%d] has already been delivered", task.ID)
|
|
|
|
continue
|
|
|
|
}
|
|
|
|
|
|
|
|
if err := Deliver(ctx, task); err != nil {
|
|
|
|
log.Error("Unable to deliver webhook task[%d]: %v", task.ID, err)
|
2022-04-25 20:03:01 +02:00
|
|
|
}
|
|
|
|
}
|
2022-10-21 18:21:56 +02:00
|
|
|
|
2022-04-25 20:03:01 +02:00
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
2022-11-23 15:10:04 +01:00
|
|
|
func enqueueHookTask(taskID int64) error {
|
|
|
|
err := hookQueue.Push(taskID)
|
2022-04-25 20:03:01 +02:00
|
|
|
if err != nil && err != queue.ErrAlreadyInQueue {
|
|
|
|
return err
|
|
|
|
}
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
2021-11-10 06:13:16 +01:00
|
|
|
func checkBranch(w *webhook_model.Webhook, branch string) bool {
|
2019-11-01 23:51:22 +01:00
|
|
|
if w.BranchFilter == "" || w.BranchFilter == "*" {
|
|
|
|
return true
|
|
|
|
}
|
|
|
|
|
|
|
|
g, err := glob.Compile(w.BranchFilter)
|
|
|
|
if err != nil {
|
|
|
|
// should not really happen as BranchFilter is validated
|
|
|
|
log.Error("CheckBranch failed: %s", err)
|
|
|
|
return false
|
|
|
|
}
|
|
|
|
|
|
|
|
return g.Match(branch)
|
|
|
|
}
|
|
|
|
|
2024-03-07 23:18:38 +01:00
|
|
|
// PrepareWebhook creates a hook task and enqueues it for processing.
|
|
|
|
// The payload is saved as-is. The adjustments depending on the webhook type happen
|
|
|
|
// right before delivery, in the [Deliver] method.
|
2023-01-01 16:23:15 +01:00
|
|
|
func PrepareWebhook(ctx context.Context, w *webhook_model.Webhook, event webhook_module.HookEventType, p api.Payloader) error {
|
2021-02-11 18:34:34 +01:00
|
|
|
// Skip sending if webhooks are disabled.
|
|
|
|
if setting.DisableWebhooks {
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
2019-11-01 23:51:22 +01:00
|
|
|
for _, e := range w.EventCheckers() {
|
|
|
|
if event == e.Type {
|
|
|
|
if !e.Has() {
|
|
|
|
return nil
|
|
|
|
}
|
2021-02-22 19:54:01 +01:00
|
|
|
|
|
|
|
break
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2020-09-03 04:46:02 +02:00
|
|
|
// Avoid sending "0 new commits" to non-integration relevant webhooks (e.g. slack, discord, etc.).
|
|
|
|
// Integration webhooks (e.g. drone) still receive the required data.
|
|
|
|
if pushEvent, ok := p.(*api.PushPayload); ok &&
|
2023-01-01 16:23:15 +01:00
|
|
|
w.Type != webhook_module.GITEA && w.Type != webhook_module.GOGS &&
|
2020-09-03 04:46:02 +02:00
|
|
|
len(pushEvent.Commits) == 0 {
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
2019-11-01 23:51:22 +01:00
|
|
|
// If payload has no associated branch (e.g. it's a new tag, issue, etc.),
|
|
|
|
// branch filter has no effect.
|
|
|
|
if branch := getPayloadBranch(p); branch != "" {
|
|
|
|
if !checkBranch(w, branch) {
|
|
|
|
log.Info("Branch %q doesn't match branch filter %q, skipping", branch, w.BranchFilter)
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
2024-03-07 23:18:38 +01:00
|
|
|
payload, err := p.JSONPayload()
|
|
|
|
if err != nil {
|
|
|
|
return fmt.Errorf("JSONPayload for %s: %w", event, err)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
|
|
|
|
2022-10-21 18:21:56 +02:00
|
|
|
task, err := webhook_model.CreateHookTask(ctx, &webhook_model.HookTask{
|
2024-03-07 23:18:38 +01:00
|
|
|
HookID: w.ID,
|
|
|
|
PayloadContent: string(payload),
|
|
|
|
EventType: event,
|
|
|
|
PayloadVersion: 2,
|
2022-10-21 18:21:56 +02:00
|
|
|
})
|
|
|
|
if err != nil {
|
2024-03-07 23:18:38 +01:00
|
|
|
return fmt.Errorf("CreateHookTask for %s: %w", event, err)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
2022-10-21 18:21:56 +02:00
|
|
|
|
2022-11-23 15:10:04 +01:00
|
|
|
return enqueueHookTask(task.ID)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
// PrepareWebhooks adds new webhooks to task queue for given payload.
|
2023-01-01 16:23:15 +01:00
|
|
|
func PrepareWebhooks(ctx context.Context, source EventSource, event webhook_module.HookEventType, p api.Payloader) error {
|
2022-10-21 18:21:56 +02:00
|
|
|
owner := source.Owner
|
2019-11-02 03:35:12 +01:00
|
|
|
|
2022-10-21 18:21:56 +02:00
|
|
|
var ws []*webhook_model.Webhook
|
2019-11-01 23:51:22 +01:00
|
|
|
|
2022-10-21 18:21:56 +02:00
|
|
|
if source.Repository != nil {
|
2023-11-24 04:49:41 +01:00
|
|
|
repoHooks, err := db.Find[webhook_model.Webhook](ctx, webhook_model.ListWebhookOptions{
|
2022-10-21 18:21:56 +02:00
|
|
|
RepoID: source.Repository.ID,
|
2024-03-02 16:42:31 +01:00
|
|
|
IsActive: optional.Some(true),
|
2022-10-21 18:21:56 +02:00
|
|
|
})
|
|
|
|
if err != nil {
|
2022-10-24 21:29:17 +02:00
|
|
|
return fmt.Errorf("ListWebhooksByOpts: %w", err)
|
2022-10-21 18:21:56 +02:00
|
|
|
}
|
|
|
|
ws = append(ws, repoHooks...)
|
|
|
|
|
2022-11-19 09:12:33 +01:00
|
|
|
owner = source.Repository.MustOwner(ctx)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
|
|
|
|
2023-03-10 15:28:32 +01:00
|
|
|
// append additional webhooks of a user or organization
|
|
|
|
if owner != nil {
|
2023-11-24 04:49:41 +01:00
|
|
|
ownerHooks, err := db.Find[webhook_model.Webhook](ctx, webhook_model.ListWebhookOptions{
|
2023-03-10 15:28:32 +01:00
|
|
|
OwnerID: owner.ID,
|
2024-03-02 16:42:31 +01:00
|
|
|
IsActive: optional.Some(true),
|
2021-08-12 14:43:08 +02:00
|
|
|
})
|
2019-11-01 23:51:22 +01:00
|
|
|
if err != nil {
|
2022-10-24 21:29:17 +02:00
|
|
|
return fmt.Errorf("ListWebhooksByOpts: %w", err)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
2023-03-10 15:28:32 +01:00
|
|
|
ws = append(ws, ownerHooks...)
|
2019-11-01 23:51:22 +01:00
|
|
|
}
|
|
|
|
|
2020-03-08 23:08:05 +01:00
|
|
|
// Add any admin-defined system webhooks
|
2024-03-02 16:42:31 +01:00
|
|
|
systemHooks, err := webhook_model.GetSystemWebhooks(ctx, optional.Some(true))
|
2020-03-08 23:08:05 +01:00
|
|
|
if err != nil {
|
2022-10-24 21:29:17 +02:00
|
|
|
return fmt.Errorf("GetSystemWebhooks: %w", err)
|
2020-03-08 23:08:05 +01:00
|
|
|
}
|
|
|
|
ws = append(ws, systemHooks...)
|
|
|
|
|
2019-11-01 23:51:22 +01:00
|
|
|
if len(ws) == 0 {
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
|
|
|
for _, w := range ws {
|
2022-10-21 18:21:56 +02:00
|
|
|
if err := PrepareWebhook(ctx, w, event, p); err != nil {
|
2019-11-01 23:51:22 +01:00
|
|
|
return err
|
|
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
|
|
}
|
2022-01-05 22:00:20 +01:00
|
|
|
|
|
|
|
// ReplayHookTask replays a webhook task
|
2022-10-21 18:21:56 +02:00
|
|
|
func ReplayHookTask(ctx context.Context, w *webhook_model.Webhook, uuid string) error {
|
|
|
|
task, err := webhook_model.ReplayHookTask(ctx, w.ID, uuid)
|
2022-01-05 22:00:20 +01:00
|
|
|
if err != nil {
|
|
|
|
return err
|
|
|
|
}
|
|
|
|
|
2022-11-23 15:10:04 +01:00
|
|
|
return enqueueHookTask(task.ID)
|
2022-01-05 22:00:20 +01:00
|
|
|
}
|