2022-08-28 11:43:25 +02:00
|
|
|
// Copyright 2022 The Gitea Authors. All rights reserved.
|
2022-11-27 19:20:29 +01:00
|
|
|
// SPDX-License-Identifier: MIT
|
2022-08-28 11:43:25 +02:00
|
|
|
|
|
|
|
package templates
|
|
|
|
|
|
|
|
import (
|
2023-04-14 07:19:11 +02:00
|
|
|
"bufio"
|
2022-10-07 23:02:24 +02:00
|
|
|
"bytes"
|
2023-04-08 15:15:22 +02:00
|
|
|
"errors"
|
2022-10-07 23:02:24 +02:00
|
|
|
"fmt"
|
2023-04-08 08:21:50 +02:00
|
|
|
"io"
|
|
|
|
"net/http"
|
|
|
|
"path/filepath"
|
2022-10-07 23:02:24 +02:00
|
|
|
"regexp"
|
|
|
|
"strconv"
|
|
|
|
"strings"
|
2023-04-30 14:22:23 +02:00
|
|
|
"sync"
|
2023-04-08 08:21:50 +02:00
|
|
|
"sync/atomic"
|
2023-04-08 15:15:22 +02:00
|
|
|
texttemplate "text/template"
|
2022-08-28 11:43:25 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
"code.gitea.io/gitea/modules/assetfs"
|
2023-04-30 14:22:23 +02:00
|
|
|
"code.gitea.io/gitea/modules/graceful"
|
2022-08-28 11:43:25 +02:00
|
|
|
"code.gitea.io/gitea/modules/log"
|
|
|
|
"code.gitea.io/gitea/modules/setting"
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
"code.gitea.io/gitea/modules/templates/scopedtmpl"
|
2023-04-08 15:15:22 +02:00
|
|
|
"code.gitea.io/gitea/modules/util"
|
2022-08-28 11:43:25 +02:00
|
|
|
)
|
|
|
|
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
type TemplateExecutor scopedtmpl.TemplateExecutor
|
|
|
|
|
2023-04-08 08:21:50 +02:00
|
|
|
type HTMLRender struct {
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
templates atomic.Pointer[scopedtmpl.ScopedTemplate]
|
2023-04-08 08:21:50 +02:00
|
|
|
}
|
|
|
|
|
2023-04-30 14:22:23 +02:00
|
|
|
var (
|
|
|
|
htmlRender *HTMLRender
|
|
|
|
htmlRenderOnce sync.Once
|
|
|
|
)
|
|
|
|
|
2023-04-08 15:15:22 +02:00
|
|
|
var ErrTemplateNotInitialized = errors.New("template system is not initialized, check your log for errors")
|
|
|
|
|
2023-04-08 08:21:50 +02:00
|
|
|
func (h *HTMLRender) HTML(w io.Writer, status int, name string, data interface{}) error {
|
|
|
|
if respWriter, ok := w.(http.ResponseWriter); ok {
|
|
|
|
if respWriter.Header().Get("Content-Type") == "" {
|
|
|
|
respWriter.Header().Set("Content-Type", "text/html; charset=utf-8")
|
2022-08-28 11:43:25 +02:00
|
|
|
}
|
2023-04-08 08:21:50 +02:00
|
|
|
respWriter.WriteHeader(status)
|
|
|
|
}
|
2023-04-08 15:15:22 +02:00
|
|
|
t, err := h.TemplateLookup(name)
|
|
|
|
if err != nil {
|
|
|
|
return texttemplate.ExecError{Name: name, Err: err}
|
|
|
|
}
|
|
|
|
return t.Execute(w, data)
|
2023-04-08 08:21:50 +02:00
|
|
|
}
|
|
|
|
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
func (h *HTMLRender) TemplateLookup(name string) (TemplateExecutor, error) {
|
2023-04-08 15:15:22 +02:00
|
|
|
tmpls := h.templates.Load()
|
|
|
|
if tmpls == nil {
|
|
|
|
return nil, ErrTemplateNotInitialized
|
|
|
|
}
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
|
2023-04-30 14:22:23 +02:00
|
|
|
return tmpls.Executor(name, NewFuncMap())
|
2023-04-08 08:21:50 +02:00
|
|
|
}
|
|
|
|
|
|
|
|
func (h *HTMLRender) CompileTemplates() error {
|
2023-04-12 12:16:45 +02:00
|
|
|
assets := AssetFS()
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
extSuffix := ".tmpl"
|
|
|
|
tmpls := scopedtmpl.NewScopedTemplate()
|
2023-04-30 14:22:23 +02:00
|
|
|
tmpls.Funcs(NewFuncMap())
|
2023-04-12 12:16:45 +02:00
|
|
|
files, err := ListWebTemplateAssetNames(assets)
|
|
|
|
if err != nil {
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
for _, file := range files {
|
|
|
|
if !strings.HasSuffix(file, extSuffix) {
|
2023-04-08 15:56:50 +02:00
|
|
|
continue
|
|
|
|
}
|
2023-04-12 12:16:45 +02:00
|
|
|
name := strings.TrimSuffix(file, extSuffix)
|
2023-04-08 08:21:50 +02:00
|
|
|
tmpl := tmpls.New(filepath.ToSlash(name))
|
2023-04-12 12:16:45 +02:00
|
|
|
buf, err := assets.ReadFile(file)
|
2023-04-08 08:21:50 +02:00
|
|
|
if err != nil {
|
|
|
|
return err
|
|
|
|
}
|
|
|
|
if _, err = tmpl.Parse(string(buf)); err != nil {
|
|
|
|
return err
|
|
|
|
}
|
|
|
|
}
|
Make HTML template functions support context (#24056)
# Background
Golang template is not friendly for large projects, and Golang template
team is quite slow, related:
* `https://github.com/golang/go/issues/54450`
Without upstream support, we can also have our solution to make HTML
template functions support context.
It helps a lot, the above Golang template issue `#54450` explains a lot:
1. It makes `{{Locale.Tr}}` could be used in any template, without
passing unclear `(dict "root" . )` anymore.
2. More and more functions need `context`, like `avatar`, etc, we do not
need to do `(dict "Context" $.Context)` anymore.
3. Many request-related functions could be shared by parent&children
templates, like "user setting" / "system setting"
See the test `TestScopedTemplateSetFuncMap`, one template set, two
`Execute` calls with different `CtxFunc`.
# The Solution
Instead of waiting for upstream, this PR re-uses the escaped HTML
template trees, use `AddParseTree` to add related templates/trees to a
new template instance, then the new template instance can have its own
FuncMap , the function calls in the template trees will always use the
new template's FuncMap.
`template.New` / `template.AddParseTree` / `adding-FuncMap` are all
quite fast, so the performance is not affected.
The details:
1. Make a new `html/template/Template` for `all` templates
2. Add template code to the `all` template
3. Freeze the `all` template, reset its exec func map, it shouldn't
execute any template.
4. When a router wants to render a template by its `name`
1. Find the `name` in `all`
2. Find all its related sub templates
3. Escape all related templates (just like what the html template
package does)
4. Add the escaped parse-trees of related templates into a new (scoped)
`text/template/Template`
5. Add context-related func map into the new (scoped) text template
6. Execute the new (scoped) text template
7. To improve performance, the escaped templates are cached to `template
sets`
# FAQ
## There is a `unsafe` call, is this PR unsafe?
This PR is safe. Golang has strict language definition, it's safe to do
so: https://pkg.go.dev/unsafe#Pointer (1) Conversion of a *T1 to Pointer
to *T2
## What if Golang template supports such feature in the future?
The public structs/interfaces/functions introduced by this PR is quite
simple, the code of `HTMLRender` is not changed too much. It's very easy
to switch to the official mechanism if there would be one.
## Does this PR change the template execution behavior?
No, see the tests (welcome to design more tests if it's necessary)
---------
Co-authored-by: silverwind <me@silverwind.io>
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-04-20 10:08:58 +02:00
|
|
|
tmpls.Freeze()
|
2023-04-08 08:21:50 +02:00
|
|
|
h.templates.Store(tmpls)
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
2023-04-30 14:22:23 +02:00
|
|
|
// HTMLRenderer init once and returns the globally shared html renderer
|
|
|
|
func HTMLRenderer() *HTMLRender {
|
|
|
|
htmlRenderOnce.Do(initHTMLRenderer)
|
|
|
|
return htmlRender
|
|
|
|
}
|
2022-08-28 11:43:25 +02:00
|
|
|
|
2023-04-30 14:22:23 +02:00
|
|
|
func initHTMLRenderer() {
|
2022-08-28 11:43:25 +02:00
|
|
|
rendererType := "static"
|
|
|
|
if !setting.IsProd {
|
|
|
|
rendererType = "auto-reloading"
|
|
|
|
}
|
2023-04-30 14:22:23 +02:00
|
|
|
log.Debug("Creating %s HTML Renderer", rendererType)
|
2022-08-28 11:43:25 +02:00
|
|
|
|
2023-04-30 14:22:23 +02:00
|
|
|
htmlRender = &HTMLRender{}
|
|
|
|
if err := htmlRender.CompileTemplates(); err != nil {
|
2023-04-14 07:19:11 +02:00
|
|
|
p := &templateErrorPrettier{assets: AssetFS()}
|
|
|
|
wrapFatal(p.handleFuncNotDefinedError(err))
|
|
|
|
wrapFatal(p.handleUnexpectedOperandError(err))
|
|
|
|
wrapFatal(p.handleExpectedEndError(err))
|
|
|
|
wrapFatal(p.handleGenericTemplateError(err))
|
|
|
|
log.Fatal("HTMLRenderer CompileTemplates error: %v", err)
|
2023-04-08 08:21:50 +02:00
|
|
|
}
|
2023-04-30 14:22:23 +02:00
|
|
|
|
2022-08-28 11:43:25 +02:00
|
|
|
if !setting.IsProd {
|
2023-04-30 14:22:23 +02:00
|
|
|
go AssetFS().WatchLocalChanges(graceful.GetManager().ShutdownContext(), func() {
|
|
|
|
if err := htmlRender.CompileTemplates(); err != nil {
|
2023-04-12 12:16:45 +02:00
|
|
|
log.Error("Template error: %v\n%s", err, log.Stack(2))
|
|
|
|
}
|
2022-08-28 11:43:25 +02:00
|
|
|
})
|
|
|
|
}
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func wrapFatal(msg string) {
|
|
|
|
if msg == "" {
|
2022-10-07 23:02:24 +02:00
|
|
|
return
|
|
|
|
}
|
Rewrite logger system (#24726)
## ⚠️ Breaking
The `log.<mode>.<logger>` style config has been dropped. If you used it,
please check the new config manual & app.example.ini to make your
instance output logs as expected.
Although many legacy options still work, it's encouraged to upgrade to
the new options.
The SMTP logger is deleted because SMTP is not suitable to collect logs.
If you have manually configured Gitea log options, please confirm the
logger system works as expected after upgrading.
## Description
Close #12082 and maybe more log-related issues, resolve some related
FIXMEs in old code (which seems unfixable before)
Just like rewriting queue #24505 : make code maintainable, clear legacy
bugs, and add the ability to support more writers (eg: JSON, structured
log)
There is a new document (with examples): `logging-config.en-us.md`
This PR is safer than the queue rewriting, because it's just for
logging, it won't break other logic.
## The old problems
The logging system is quite old and difficult to maintain:
* Unclear concepts: Logger, NamedLogger, MultiChannelledLogger,
SubLogger, EventLogger, WriterLogger etc
* Some code is diffuclt to konw whether it is right:
`log.DelNamedLogger("console")` vs `log.DelNamedLogger(log.DEFAULT)` vs
`log.DelLogger("console")`
* The old system heavily depends on ini config system, it's difficult to
create new logger for different purpose, and it's very fragile.
* The "color" trick is difficult to use and read, many colors are
unnecessary, and in the future structured log could help
* It's difficult to add other log formats, eg: JSON format
* The log outputer doesn't have full control of its goroutine, it's
difficult to make outputer have advanced behaviors
* The logs could be lost in some cases: eg: no Fatal error when using
CLI.
* Config options are passed by JSON, which is quite fragile.
* INI package makes the KEY in `[log]` section visible in `[log.sub1]`
and `[log.sub1.subA]`, this behavior is quite fragile and would cause
more unclear problems, and there is no strong requirement to support
`log.<mode>.<logger>` syntax.
## The new design
See `logger.go` for documents.
## Screenshot
<details>


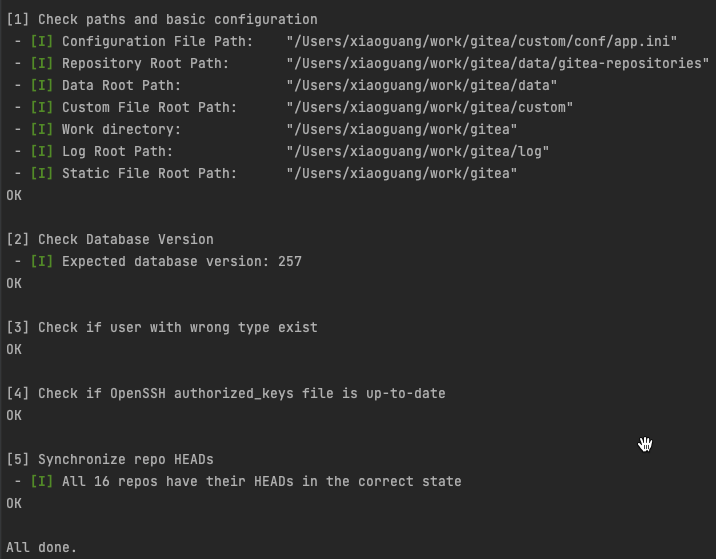
</details>
## TODO
* [x] add some new tests
* [x] fix some tests
* [x] test some sub-commands (manually ....)
---------
Co-authored-by: Jason Song <i@wolfogre.com>
Co-authored-by: delvh <dev.lh@web.de>
Co-authored-by: Giteabot <teabot@gitea.io>
2023-05-22 00:35:11 +02:00
|
|
|
log.Fatal("Unable to compile templates, %s", msg)
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
type templateErrorPrettier struct {
|
|
|
|
assets *assetfs.LayeredFS
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var reGenericTemplateError = regexp.MustCompile(`^template: (.*):([0-9]+): (.*)`)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) handleGenericTemplateError(err error) string {
|
|
|
|
groups := reGenericTemplateError.FindStringSubmatch(err.Error())
|
2022-10-07 23:02:24 +02:00
|
|
|
if len(groups) != 4 {
|
2023-04-14 07:19:11 +02:00
|
|
|
return ""
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
tmplName, lineStr, message := groups[1], groups[2], groups[3]
|
|
|
|
return p.makeDetailedError(message, tmplName, lineStr, -1, "")
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var reFuncNotDefinedError = regexp.MustCompile(`^template: (.*):([0-9]+): (function "(.*)" not defined)`)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) handleFuncNotDefinedError(err error) string {
|
|
|
|
groups := reFuncNotDefinedError.FindStringSubmatch(err.Error())
|
|
|
|
if len(groups) != 5 {
|
|
|
|
return ""
|
|
|
|
}
|
|
|
|
tmplName, lineStr, message, funcName := groups[1], groups[2], groups[3], groups[4]
|
|
|
|
funcName, _ = strconv.Unquote(`"` + funcName + `"`)
|
|
|
|
return p.makeDetailedError(message, tmplName, lineStr, -1, funcName)
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var reUnexpectedOperandError = regexp.MustCompile(`^template: (.*):([0-9]+): (unexpected "(.*)" in operand)`)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) handleUnexpectedOperandError(err error) string {
|
|
|
|
groups := reUnexpectedOperandError.FindStringSubmatch(err.Error())
|
|
|
|
if len(groups) != 5 {
|
|
|
|
return ""
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
tmplName, lineStr, message, unexpected := groups[1], groups[2], groups[3], groups[4]
|
|
|
|
unexpected, _ = strconv.Unquote(`"` + unexpected + `"`)
|
|
|
|
return p.makeDetailedError(message, tmplName, lineStr, -1, unexpected)
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var reExpectedEndError = regexp.MustCompile(`^template: (.*):([0-9]+): (expected end; found (.*))`)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) handleExpectedEndError(err error) string {
|
|
|
|
groups := reExpectedEndError.FindStringSubmatch(err.Error())
|
|
|
|
if len(groups) != 5 {
|
|
|
|
return ""
|
|
|
|
}
|
|
|
|
tmplName, lineStr, message, unexpected := groups[1], groups[2], groups[3], groups[4]
|
|
|
|
return p.makeDetailedError(message, tmplName, lineStr, -1, unexpected)
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var (
|
|
|
|
reTemplateExecutingError = regexp.MustCompile(`^template: (.*):([1-9][0-9]*):([1-9][0-9]*): (executing .*)`)
|
|
|
|
reTemplateExecutingErrorMsg = regexp.MustCompile(`^executing "(.*)" at <(.*)>: `)
|
|
|
|
)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) handleTemplateRenderingError(err error) string {
|
|
|
|
if groups := reTemplateExecutingError.FindStringSubmatch(err.Error()); len(groups) > 0 {
|
|
|
|
tmplName, lineStr, posStr, msgPart := groups[1], groups[2], groups[3], groups[4]
|
|
|
|
target := ""
|
|
|
|
if groups = reTemplateExecutingErrorMsg.FindStringSubmatch(msgPart); len(groups) > 0 {
|
|
|
|
target = groups[2]
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
return p.makeDetailedError(msgPart, tmplName, lineStr, posStr, target)
|
|
|
|
} else if execErr, ok := err.(texttemplate.ExecError); ok {
|
|
|
|
layerName := p.assets.GetFileLayerName(execErr.Name + ".tmpl")
|
|
|
|
return fmt.Sprintf("asset from: %s, %s", layerName, err.Error())
|
|
|
|
} else {
|
|
|
|
return err.Error()
|
|
|
|
}
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func HandleTemplateRenderingError(err error) string {
|
|
|
|
p := &templateErrorPrettier{assets: AssetFS()}
|
|
|
|
return p.handleTemplateRenderingError(err)
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
const dashSeparator = "----------------------------------------------------------------------"
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
func (p *templateErrorPrettier) makeDetailedError(errMsg, tmplName string, lineNum, posNum any, target string) string {
|
|
|
|
code, layer, err := p.assets.ReadLayeredFile(tmplName + ".tmpl")
|
|
|
|
if err != nil {
|
|
|
|
return fmt.Sprintf("template error: %s, and unable to find template file %q", errMsg, tmplName)
|
|
|
|
}
|
|
|
|
line, err := util.ToInt64(lineNum)
|
|
|
|
if err != nil {
|
|
|
|
return fmt.Sprintf("template error: %s, unable to parse template %q line number %q", errMsg, tmplName, lineNum)
|
|
|
|
}
|
|
|
|
pos, err := util.ToInt64(posNum)
|
|
|
|
if err != nil {
|
|
|
|
return fmt.Sprintf("template error: %s, unable to parse template %q pos number %q", errMsg, tmplName, posNum)
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
detail := extractErrorLine(code, int(line), int(pos), target)
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
var msg string
|
|
|
|
if pos >= 0 {
|
|
|
|
msg = fmt.Sprintf("template error: %s:%s:%d:%d : %s", layer, tmplName, line, pos, errMsg)
|
|
|
|
} else {
|
|
|
|
msg = fmt.Sprintf("template error: %s:%s:%d : %s", layer, tmplName, line, errMsg)
|
2023-03-20 21:56:48 +01:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
return msg + "\n" + dashSeparator + "\n" + detail + "\n" + dashSeparator
|
|
|
|
}
|
|
|
|
|
|
|
|
func extractErrorLine(code []byte, lineNum, posNum int, target string) string {
|
|
|
|
b := bufio.NewReader(bytes.NewReader(code))
|
|
|
|
var line []byte
|
|
|
|
var err error
|
|
|
|
for i := 0; i < lineNum; i++ {
|
|
|
|
if line, err = b.ReadBytes('\n'); err != nil {
|
|
|
|
if i == lineNum-1 && errors.Is(err, io.EOF) {
|
|
|
|
err = nil
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
break
|
2023-03-20 21:56:48 +01:00
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
}
|
|
|
|
if err != nil {
|
|
|
|
return fmt.Sprintf("unable to find target line %d", lineNum)
|
|
|
|
}
|
2022-10-07 23:02:24 +02:00
|
|
|
|
2023-04-14 07:19:11 +02:00
|
|
|
line = bytes.TrimRight(line, "\r\n")
|
|
|
|
var indicatorLine []byte
|
|
|
|
targetBytes := []byte(target)
|
|
|
|
targetLen := len(targetBytes)
|
|
|
|
for i := 0; i < len(line); {
|
|
|
|
if posNum == -1 && target != "" && bytes.HasPrefix(line[i:], targetBytes) {
|
|
|
|
for j := 0; j < targetLen && i < len(line); j++ {
|
|
|
|
indicatorLine = append(indicatorLine, '^')
|
|
|
|
i++
|
|
|
|
}
|
|
|
|
} else if i == posNum {
|
|
|
|
indicatorLine = append(indicatorLine, '^')
|
|
|
|
i++
|
|
|
|
} else {
|
|
|
|
if line[i] == '\t' {
|
|
|
|
indicatorLine = append(indicatorLine, '\t')
|
|
|
|
} else {
|
|
|
|
indicatorLine = append(indicatorLine, ' ')
|
|
|
|
}
|
|
|
|
i++
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|
|
|
|
}
|
2023-04-14 07:19:11 +02:00
|
|
|
// if the indicatorLine only contains spaces, trim it together
|
|
|
|
return strings.TrimRight(string(line)+"\n"+string(indicatorLine), " \t\r\n")
|
2022-10-07 23:02:24 +02:00
|
|
|
}
|