2022-01-28 22:00:11 +01:00
import $ from 'jquery' ;
2021-10-16 19:28:04 +02:00
import {
2022-12-23 17:03:11 +01:00
initRepoIssueBranchSelect , initRepoIssueCodeCommentCancel , initRepoIssueCommentDelete ,
initRepoIssueComments , initRepoIssueDependencyDelete , initRepoIssueReferenceIssue ,
2023-04-03 12:06:57 +02:00
initRepoIssueTitleEdit , initRepoIssueWipToggle ,
2023-05-09 00:22:52 +02:00
initRepoPullRequestUpdate , updateIssuesMeta , handleReply , initIssueTemplateCommentEditors , initSingleCommentEditor ,
2021-10-16 19:28:04 +02:00
} from './repo-issue.js' ;
2022-01-07 02:18:52 +01:00
import { initUnicodeEscapeButton } from './repo-unicode-escape.js' ;
2021-10-16 19:28:04 +02:00
import { svg } from '../svg.js' ;
import { htmlEscape } from 'escape-goat' ;
2023-03-14 10:51:20 +01:00
import { initRepoBranchTagSelector } from '../components/RepoBranchTagSelector.vue' ;
2021-10-16 19:28:04 +02:00
import {
2022-12-23 17:03:11 +01:00
initRepoCloneLink , initRepoCommonBranchOrTagDropdown , initRepoCommonFilterSearchDropdown ,
2021-10-16 19:28:04 +02:00
} from './repo-common.js' ;
2022-11-11 18:02:50 +01:00
import { initCitationFileCopyContent } from './citation.js' ;
2021-10-16 19:28:04 +02:00
import { initCompLabelEdit } from './comp/LabelEdit.js' ;
import { initRepoDiffConversationNav } from './repo-diff.js' ;
2022-12-23 17:03:11 +01:00
import { createDropzone } from './dropzone.js' ;
2021-10-16 19:28:04 +02:00
import { initCommentContent , initMarkupContent } from '../markup/content.js' ;
import { initCompReactionSelector } from './comp/ReactionSelector.js' ;
import { initRepoSettingBranches } from './repo-settings.js' ;
2022-12-23 17:03:11 +01:00
import { initRepoPullRequestMergeForm } from './repo-issue-pr-form.js' ;
2023-11-02 15:49:02 +01:00
import { initRepoPullRequestCommitStatus } from './repo-issue-pr-status.js' ;
2023-02-19 05:06:14 +01:00
import { hideElem , showElem } from '../utils/dom.js' ;
2023-04-03 12:06:57 +02:00
import { getComboMarkdownEditor , initComboMarkdownEditor } from './comp/ComboMarkdownEditor.js' ;
Append `(comment)` when a link points at a comment rather than the whole issue (#23734)
Close #23671
For the feature mentioned above, this PR append ' (comment)' to the
rendered html if it is a hashcomment.
After the PR, type in the following
```
pull request from other repo:
http://localhost:3000/testOrg/testOrgRepo/pulls/2
pull request from this repo:
http://localhost:3000/aaa/testA/pulls/2
issue comment from this repo:
http://localhost:3000/aaa/testA/issues/1#issuecomment-18
http://localhost:3000/aaa/testA/pulls/2#issue-9
issue comment from other repo:
http://localhost:3000/testOrg/testOrgRepo/pulls/2#issuecomment-24
http://localhost:3000/testOrg/testOrgRepo/pulls/2#issue
```
Gives:
<img width="687" alt="截屏2023-03-27 13 53 06"
src="https://user-images.githubusercontent.com/17645053/227852387-2b218e0d-3468-4d90-ad81-d702ddd17fd2.png">
Other than the above feature, this PR also includes two other changes:
1 Right now, the render of links from file changed tab in pull request
might not be very proper, for example, if type in the following. (not
sure if this is an issue or design, if not an issue, I will revert the
changes). example on
[try.gitea.io](https://try.gitea.io/HesterG/testrepo/pulls/1)
```
https://try.gitea.io/HesterG/testrepo/pulls/1/files#issuecomment-162725
https://try.gitea.io/HesterG/testrepo/pulls/1/files
```
it will render the following
<img width="899" alt="截屏2023-03-24 15 41 37"
src="https://user-images.githubusercontent.com/17645053/227456117-5eccedb7-9118-4540-929d-aee9a76de852.png">
In this PR, skip processing the link into a ref issue if it is a link
from files changed tab in pull request
After:
type in following
```
hash comment on files changed tab:
http://localhost:3000/testOrg/testOrgRepo/pulls/2/files#issuecomment-24
files changed link:
http://localhost:3000/testOrg/testOrgRepo/pulls/2/files
```
Gives
<img width="708" alt="截屏2023-03-27 22 09 02"
src="https://user-images.githubusercontent.com/17645053/227964273-5dc06c50-3713-489c-b05d-d95367d0ab0f.png">
2 Right now, after editing the comment area, there will not be tippys
attached to `ref-issue`; and no tippy attached on preview as well.
example:
https://user-images.githubusercontent.com/17645053/227850540-5ae34e2d-b1d7-4d0d-9726-7701bf825d1f.mov
In this PR, in frontend, make sure tippy is added after editing the
comment, and to the comment on preview tab
After:
https://user-images.githubusercontent.com/17645053/227853777-06f56b4c-1148-467c-b6f7-f79418e67504.mov
2023-04-03 10:02:57 +02:00
import { attachRefIssueContextPopup } from './contextpopup.js' ;
2024-03-15 19:02:43 +01:00
import { POST , GET } from '../modules/fetch.js' ;
2021-10-16 19:28:04 +02:00
2021-10-21 09:37:43 +02:00
const { csrfToken } = window . config ;
2021-10-16 19:28:04 +02:00
2023-04-03 12:06:57 +02:00
// if there are draft comments, confirm before reloading, to avoid losing comments
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
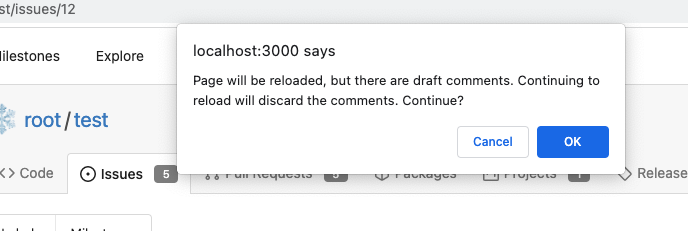
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
function reloadConfirmDraftComment ( ) {
const commentTextareas = [
2024-03-24 19:23:38 +01:00
document . querySelector ( '.edit-content-zone:not(.tw-hidden) textarea' ) ,
2023-04-03 12:06:57 +02:00
document . querySelector ( '#comment-form textarea' ) ,
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
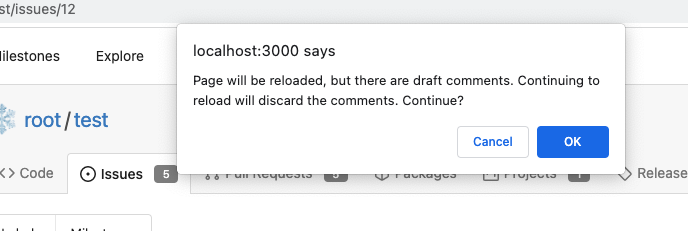
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
] ;
for ( const textarea of commentTextareas ) {
2023-04-03 12:06:57 +02:00
// Most users won't feel too sad if they lose a comment with 10 chars, they can re-type these in seconds.
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
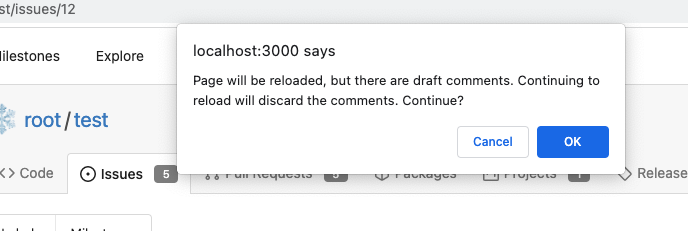
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
// But if they have typed more (like 50) chars and the comment is lost, they will be very unhappy.
2023-04-03 12:06:57 +02:00
if ( textarea && textarea . value . trim ( ) . length > 10 ) {
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
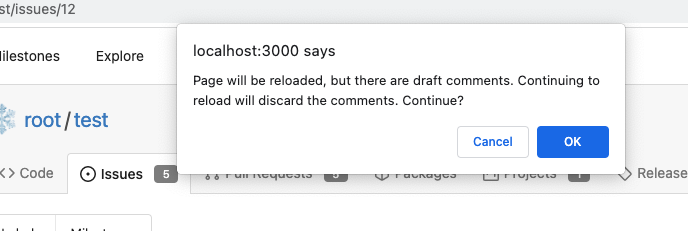
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
textarea . parentElement . scrollIntoView ( ) ;
if ( ! window . confirm ( 'Page will be reloaded, but there are draft comments. Continuing to reload will discard the comments. Continue?' ) ) {
return ;
}
break ;
}
}
window . location . reload ( ) ;
}
2021-10-16 19:28:04 +02:00
export function initRepoCommentForm ( ) {
2022-06-28 19:52:58 +02:00
const $commentForm = $ ( '.comment.form' ) ;
2024-03-25 19:37:55 +01:00
if ( ! $commentForm . length ) return ;
2021-10-16 19:28:04 +02:00
2023-05-09 00:22:52 +02:00
if ( $commentForm . find ( '.field.combo-editor-dropzone' ) . length ) {
2023-08-23 17:15:06 +02:00
// at the moment, if a form has multiple combo-markdown-editors, it must be an issue template form
2023-05-09 00:22:52 +02:00
initIssueTemplateCommentEditors ( $commentForm ) ;
2023-08-23 17:15:06 +02:00
} else if ( $commentForm . find ( '.combo-markdown-editor' ) . length ) {
// it's quite unclear about the "comment form" elements, sometimes it's for issue comment, sometimes it's for file editor/uploader message
2023-05-09 00:22:52 +02:00
initSingleCommentEditor ( $commentForm ) ;
}
2021-10-16 19:28:04 +02:00
function initBranchSelector ( ) {
const $selectBranch = $ ( '.ui.select-branch' ) ;
const $branchMenu = $selectBranch . find ( '.reference-list-menu' ) ;
const $isNewIssue = $branchMenu . hasClass ( 'new-issue' ) ;
2024-03-12 05:29:51 +01:00
$branchMenu . find ( '.item:not(.no-select)' ) . on ( 'click' , async function ( ) {
2021-10-16 19:28:04 +02:00
const selectedValue = $ ( this ) . data ( 'id' ) ;
const editMode = $ ( '#editing_mode' ) . val ( ) ;
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . data ( 'id-selector' ) ) . val ( selectedValue ) ;
2021-10-16 19:28:04 +02:00
if ( $isNewIssue ) {
$selectBranch . find ( '.ui .branch-name' ) . text ( $ ( this ) . data ( 'name' ) ) ;
return ;
}
if ( editMode === 'true' ) {
2024-03-26 20:49:38 +01:00
const form = document . getElementById ( 'update_issueref_form' ) ;
2024-03-12 05:29:51 +01:00
const params = new URLSearchParams ( ) ;
params . append ( 'ref' , selectedValue ) ;
try {
2024-03-26 20:49:38 +01:00
await POST ( form . getAttribute ( 'action' ) , { data : params } ) ;
2024-03-12 05:29:51 +01:00
window . location . reload ( ) ;
} catch ( error ) {
2024-03-15 19:02:43 +01:00
console . error ( error ) ;
2024-03-12 05:29:51 +01:00
}
2021-10-16 19:28:04 +02:00
} else if ( editMode === '' ) {
$selectBranch . find ( '.ui .branch-name' ) . text ( selectedValue ) ;
}
} ) ;
$selectBranch . find ( '.reference.column' ) . on ( 'click' , function ( ) {
2023-02-19 05:06:14 +01:00
hideElem ( $selectBranch . find ( '.scrolling.reference-list-menu' ) ) ;
2021-10-16 19:28:04 +02:00
$selectBranch . find ( '.reference .text' ) . removeClass ( 'black' ) ;
2023-02-19 05:06:14 +01:00
showElem ( $ ( $ ( this ) . data ( 'target' ) ) ) ;
2021-10-16 19:28:04 +02:00
$ ( this ) . find ( '.text' ) . addClass ( 'black' ) ;
return false ;
} ) ;
}
initBranchSelector ( ) ;
2021-11-18 17:45:00 +01:00
// List submits
2021-10-16 19:28:04 +02:00
function initListSubmits ( selector , outerSelector ) {
const $list = $ ( ` .ui. ${ outerSelector } .list ` ) ;
const $noSelect = $list . find ( '.no-select' ) ;
const $listMenu = $ ( ` . ${ selector } .menu ` ) ;
let hasUpdateAction = $listMenu . data ( 'action' ) === 'update' ;
const items = { } ;
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
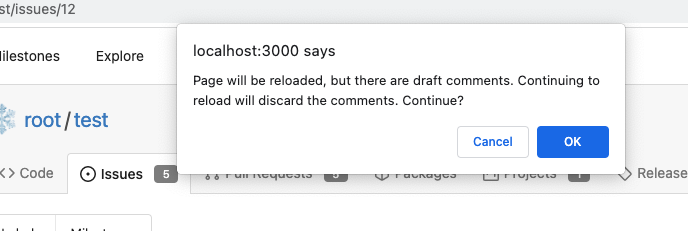
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
$ ( ` . ${ selector } ` ) . dropdown ( {
'action' : 'nothing' , // do not hide the menu if user presses Enter
fullTextSearch : 'exact' ,
async onHide ( ) {
hasUpdateAction = $listMenu . data ( 'action' ) === 'update' ; // Update the var
if ( hasUpdateAction ) {
// TODO: Add batch functionality and make this 1 network request.
const itemEntries = Object . entries ( items ) ;
for ( const [ elementId , item ] of itemEntries ) {
2021-11-16 03:21:13 +01:00
await updateIssuesMeta (
item [ 'update-url' ] ,
item . action ,
item [ 'issue-id' ] ,
elementId ,
) ;
}
Make issue meta dropdown support Enter, confirm before reloading (#23014)
As the title. Label/assignee share the same code.
* Close #22607
* Close #20727
Also:
* partially fix for #21742, now the comment reaction and menu work with
keyboard.
* partially fix for #17705, in most cases the comment won't be lost.
* partially fix for #21539
* partially fix for #20347
* partially fix for #7329
### The `Enter` support
Before, if user presses Enter, the dropdown just disappears and nothing
happens or the window reloads.
After, Enter can be used to select/deselect labels, and press Esc to
hide the dropdown to update the labels (still no way to cancel ....
maybe you can do a Cmd+R or F5 to refresh the window to discard the
changes .....)
This is only a quick patch, the UX is still not perfect, but it's much
better than before.
### The `confirm` before reloading
And more fixes for the `reload` problem, the new behaviors:
* If nothing changes (just show/hide the dropdown), then the page won't
be reloaded.
* If there are draft comments, show a confirm dialog before reloading,
to avoid losing comments.
That's the best effect can be done at the moment, unless completely
refactor these dropdown related code.
Screenshot of the confirm dialog:
<details>
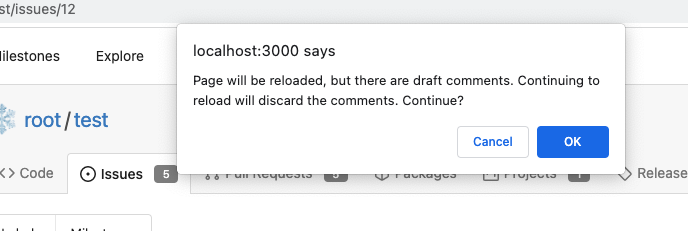
</details>
---------
Co-authored-by: Brecht Van Lommel <brecht@blender.org>
Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
2023-02-24 02:26:27 +01:00
if ( itemEntries . length ) {
reloadConfirmDraftComment ( ) ;
}
}
} ,
2021-10-16 19:28:04 +02:00
} ) ;
$listMenu . find ( '.item:not(.no-select)' ) . on ( 'click' , function ( e ) {
e . preventDefault ( ) ;
if ( $ ( this ) . hasClass ( 'ban-change' ) ) {
return false ;
}
hasUpdateAction = $listMenu . data ( 'action' ) === 'update' ; // Update the var
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
2024-03-24 18:56:02 +01:00
const clickedItem = this ; // eslint-disable-line unicorn/no-this-assignment
2024-03-26 20:49:38 +01:00
const scope = this . getAttribute ( 'data-scope' ) ;
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
$ ( this ) . parent ( ) . find ( '.item' ) . each ( function ( ) {
if ( scope ) {
// Enable only clicked item for scoped labels
2024-03-26 20:49:38 +01:00
if ( this . getAttribute ( 'data-scope' ) !== scope ) {
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
return true ;
2021-10-16 19:28:04 +02:00
}
2024-03-24 18:56:02 +01:00
if ( this !== clickedItem && ! $ ( this ) . hasClass ( 'checked' ) ) {
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
return true ;
}
2024-03-24 18:56:02 +01:00
} else if ( this !== clickedItem ) {
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
// Toggle for other labels
return true ;
2021-10-16 19:28:04 +02:00
}
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
if ( $ ( this ) . hasClass ( 'checked' ) ) {
$ ( this ) . removeClass ( 'checked' ) ;
2024-03-05 06:29:32 +01:00
$ ( this ) . find ( '.octicon-check' ) . addClass ( 'tw-invisible' ) ;
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
if ( hasUpdateAction ) {
if ( ! ( $ ( this ) . data ( 'id' ) in items ) ) {
items [ $ ( this ) . data ( 'id' ) ] = {
'update-url' : $listMenu . data ( 'update-url' ) ,
action : 'detach' ,
'issue-id' : $listMenu . data ( 'issue-id' ) ,
} ;
} else {
delete items [ $ ( this ) . data ( 'id' ) ] ;
}
}
} else {
$ ( this ) . addClass ( 'checked' ) ;
2024-03-05 06:29:32 +01:00
$ ( this ) . find ( '.octicon-check' ) . removeClass ( 'tw-invisible' ) ;
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
if ( hasUpdateAction ) {
if ( ! ( $ ( this ) . data ( 'id' ) in items ) ) {
items [ $ ( this ) . data ( 'id' ) ] = {
'update-url' : $listMenu . data ( 'update-url' ) ,
action : 'attach' ,
'issue-id' : $listMenu . data ( 'issue-id' ) ,
} ;
} else {
delete items [ $ ( this ) . data ( 'id' ) ] ;
}
2021-10-16 19:28:04 +02:00
}
}
Scoped labels (#22585)
Add a new "exclusive" option per label. This makes it so that when the
label is named `scope/name`, no other label with the same `scope/`
prefix can be set on an issue.
The scope is determined by the last occurence of `/`, so for example
`scope/alpha/name` and `scope/beta/name` are considered to be in
different scopes and can coexist.
Exclusive scopes are not enforced by any database rules, however they
are enforced when editing labels at the models level, automatically
removing any existing labels in the same scope when either attaching a
new label or replacing all labels.
In menus use a circle instead of checkbox to indicate they function as
radio buttons per scope. Issue filtering by label ensures that only a
single scoped label is selected at a time. Clicking with alt key can be
used to remove a scoped label, both when editing individual issues and
batch editing.
Label rendering refactor for consistency and code simplification:
* Labels now consistently have the same shape, emojis and tooltips
everywhere. This includes the label list and label assignment menus.
* In label list, show description below label same as label menus.
* Don't use exactly black/white text colors to look a bit nicer.
* Simplify text color computation. There is no point computing luminance
in linear color space, as this is a perceptual problem and sRGB is
closer to perceptually linear.
* Increase height of label assignment menus to show more labels. Showing
only 3-4 labels at a time leads to a lot of scrolling.
* Render all labels with a new RenderLabel template helper function.
Label creation and editing in multiline modal menu:
* Change label creation to open a modal menu like label editing.
* Change menu layout to place name, description and colors on separate
lines.
* Don't color cancel button red in label editing modal menu.
* Align text to the left in model menu for better readability and
consistent with settings layout elsewhere.
Custom exclusive scoped label rendering:
* Display scoped label prefix and suffix with slightly darker and
lighter background color respectively, and a slanted edge between them
similar to the `/` symbol.
* In menus exclusive labels are grouped with a divider line.
---------
Co-authored-by: Yarden Shoham <hrsi88@gmail.com>
Co-authored-by: Lauris BH <lauris@nix.lv>
2023-02-18 20:17:39 +01:00
} ) ;
2021-10-16 19:28:04 +02:00
// TODO: Which thing should be done for choosing review requests
// to make chosen items be shown on time here?
if ( selector === 'select-reviewers-modify' || selector === 'select-assignees-modify' ) {
return false ;
}
const listIds = [ ] ;
$ ( this ) . parent ( ) . find ( '.item' ) . each ( function ( ) {
if ( $ ( this ) . hasClass ( 'checked' ) ) {
listIds . push ( $ ( this ) . data ( 'id' ) ) ;
2024-03-24 19:23:38 +01:00
$ ( $ ( this ) . data ( 'id-selector' ) ) . removeClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
} else {
2024-03-24 19:23:38 +01:00
$ ( $ ( this ) . data ( 'id-selector' ) ) . addClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
}
} ) ;
2024-03-25 19:37:55 +01:00
if ( ! listIds . length ) {
2024-03-24 19:23:38 +01:00
$noSelect . removeClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
} else {
2024-03-24 19:23:38 +01:00
$noSelect . addClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
}
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . parent ( ) . data ( 'id' ) ) . val ( listIds . join ( ',' ) ) ;
2021-10-16 19:28:04 +02:00
return false ;
} ) ;
$listMenu . find ( '.no-select.item' ) . on ( 'click' , function ( e ) {
e . preventDefault ( ) ;
if ( hasUpdateAction ) {
2024-02-16 22:41:23 +01:00
( async ( ) => {
await updateIssuesMeta (
$listMenu . data ( 'update-url' ) ,
'clear' ,
$listMenu . data ( 'issue-id' ) ,
'' ,
) ;
reloadConfirmDraftComment ( ) ;
} ) ( ) ;
2021-10-16 19:28:04 +02:00
}
$ ( this ) . parent ( ) . find ( '.item' ) . each ( function ( ) {
$ ( this ) . removeClass ( 'checked' ) ;
2024-03-05 06:29:32 +01:00
$ ( this ) . find ( '.octicon-check' ) . addClass ( 'tw-invisible' ) ;
2021-10-16 19:28:04 +02:00
} ) ;
if ( selector === 'select-reviewers-modify' || selector === 'select-assignees-modify' ) {
return false ;
}
$list . find ( '.item' ) . each ( function ( ) {
2024-03-24 19:23:38 +01:00
$ ( this ) . addClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
} ) ;
2024-03-24 19:23:38 +01:00
$noSelect . removeClass ( 'tw-hidden' ) ;
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . parent ( ) . data ( 'id' ) ) . val ( '' ) ;
2021-10-16 19:28:04 +02:00
} ) ;
}
// Init labels and assignees
initListSubmits ( 'select-label' , 'labels' ) ;
initListSubmits ( 'select-assignees' , 'assignees' ) ;
initListSubmits ( 'select-assignees-modify' , 'assignees' ) ;
initListSubmits ( 'select-reviewers-modify' , 'assignees' ) ;
function selectItem ( select _id , input _id ) {
const $menu = $ ( ` ${ select _id } .menu ` ) ;
const $list = $ ( ` .ui ${ select _id } .list ` ) ;
const hasUpdateAction = $menu . data ( 'action' ) === 'update' ;
$menu . find ( '.item:not(.no-select)' ) . on ( 'click' , function ( ) {
$ ( this ) . parent ( ) . find ( '.item' ) . each ( function ( ) {
$ ( this ) . removeClass ( 'selected active' ) ;
} ) ;
$ ( this ) . addClass ( 'selected active' ) ;
if ( hasUpdateAction ) {
2024-02-16 22:41:23 +01:00
( async ( ) => {
await updateIssuesMeta (
$menu . data ( 'update-url' ) ,
'' ,
$menu . data ( 'issue-id' ) ,
$ ( this ) . data ( 'id' ) ,
) ;
reloadConfirmDraftComment ( ) ;
} ) ( ) ;
2021-10-16 19:28:04 +02:00
}
let icon = '' ;
if ( input _id === '#milestone_id' ) {
Migrate margin and padding helpers to tailwind (#30043)
This will conclude the refactor of 1:1 class replacements to tailwind,
except `gt-hidden`. Commands ran:
```bash
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-0#tw-$1$2-0#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-1#tw-$1$2-0.5#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-2#tw-$1$2-1#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-3#tw-$1$2-2#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-4#tw-$1$2-4#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-5#tw-$1$2-8#g' {web_src/js,templates,routers,services}/**/*
```
2024-03-24 17:42:49 +01:00
icon = svg ( 'octicon-milestone' , 18 , 'tw-mr-2' ) ;
2021-10-16 19:28:04 +02:00
} else if ( input _id === '#project_id' ) {
Migrate margin and padding helpers to tailwind (#30043)
This will conclude the refactor of 1:1 class replacements to tailwind,
except `gt-hidden`. Commands ran:
```bash
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-0#tw-$1$2-0#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-1#tw-$1$2-0.5#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-2#tw-$1$2-1#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-3#tw-$1$2-2#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-4#tw-$1$2-4#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-5#tw-$1$2-8#g' {web_src/js,templates,routers,services}/**/*
```
2024-03-24 17:42:49 +01:00
icon = svg ( 'octicon-project' , 18 , 'tw-mr-2' ) ;
2021-10-16 19:28:04 +02:00
} else if ( input _id === '#assignee_id' ) {
Migrate margin and padding helpers to tailwind (#30043)
This will conclude the refactor of 1:1 class replacements to tailwind,
except `gt-hidden`. Commands ran:
```bash
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-0#tw-$1$2-0#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-1#tw-$1$2-0.5#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-2#tw-$1$2-1#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-3#tw-$1$2-2#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-4#tw-$1$2-4#g' {web_src/js,templates,routers,services}/**/*
perl -p -i -e 's#gt-(p|m)([lrtbxy])?-5#tw-$1$2-8#g' {web_src/js,templates,routers,services}/**/*
```
2024-03-24 17:42:49 +01:00
icon = ` <img class="ui avatar image tw-mr-2" alt="avatar" src= ${ $ ( this ) . data ( 'avatar' ) } > ` ;
2021-10-16 19:28:04 +02:00
}
$list . find ( '.selected' ) . html ( `
< a class = "item muted sidebar-item-link" href = $ { $ ( this ) . data ( 'href' ) } >
$ { icon }
$ { htmlEscape ( $ ( this ) . text ( ) ) }
< / a >
` );
2024-03-24 19:23:38 +01:00
$ ( ` .ui ${ select _id } .list .no-select ` ) . addClass ( 'tw-hidden' ) ;
2021-10-16 19:28:04 +02:00
$ ( input _id ) . val ( $ ( this ) . data ( 'id' ) ) ;
} ) ;
$menu . find ( '.no-select.item' ) . on ( 'click' , function ( ) {
$ ( this ) . parent ( ) . find ( '.item:not(.no-select)' ) . each ( function ( ) {
$ ( this ) . removeClass ( 'selected active' ) ;
} ) ;
if ( hasUpdateAction ) {
2024-02-16 22:41:23 +01:00
( async ( ) => {
await updateIssuesMeta (
$menu . data ( 'update-url' ) ,
'' ,
$menu . data ( 'issue-id' ) ,
$ ( this ) . data ( 'id' ) ,
) ;
reloadConfirmDraftComment ( ) ;
} ) ( ) ;
2021-10-16 19:28:04 +02:00
}
$list . find ( '.selected' ) . html ( '' ) ;
2024-03-24 19:23:38 +01:00
$list . find ( '.no-select' ) . removeClass ( 'tw-hidden' ) ;
2022-01-16 12:19:26 +01:00
$ ( input _id ) . val ( '' ) ;
2021-10-16 19:28:04 +02:00
} ) ;
}
// Milestone, Assignee, Project
selectItem ( '.select-project' , '#project_id' ) ;
selectItem ( '.select-milestone' , '#milestone_id' ) ;
selectItem ( '.select-assignee' , '#assignee_id' ) ;
}
2021-11-18 17:45:00 +01:00
async function onEditContent ( event ) {
event . preventDefault ( ) ;
2022-01-05 13:17:25 +01:00
2024-03-26 20:49:38 +01:00
const segment = this . closest ( '.header' ) . nextElementSibling ;
const editContentZone = segment . querySelector ( '.edit-content-zone' ) ;
const renderContent = segment . querySelector ( '.render-content' ) ;
const rawContent = segment . querySelector ( '.raw-content' ) ;
2021-11-18 17:45:00 +01:00
2023-04-03 12:06:57 +02:00
let comboMarkdownEditor ;
2024-03-26 20:49:38 +01:00
/ * *
* @ param { HTMLElement } dropzone
* /
const setupDropzone = async ( dropzone ) => {
if ( ! dropzone ) return null ;
2023-04-03 12:06:57 +02:00
2023-04-20 08:39:44 +02:00
let disableRemovedfileEvent = false ; // when resetting the dropzone (removeAllFiles), disable the "removedfile" event
let fileUuidDict = { } ; // to record: if a comment has been saved, then the uploaded files won't be deleted from server when clicking the Remove in the dropzone
2024-03-26 20:49:38 +01:00
const dz = await createDropzone ( dropzone , {
url : dropzone . getAttribute ( 'data-upload-url' ) ,
2023-04-03 12:06:57 +02:00
headers : { 'X-Csrf-Token' : csrfToken } ,
2024-03-26 20:49:38 +01:00
maxFiles : dropzone . getAttribute ( 'data-max-file' ) ,
maxFilesize : dropzone . getAttribute ( 'data-max-size' ) ,
acceptedFiles : [ '*/*' , '' ] . includes ( dropzone . getAttribute ( 'data-accepts' ) ) ? null : dropzone . getAttribute ( 'data-accepts' ) ,
2023-04-03 12:06:57 +02:00
addRemoveLinks : true ,
2024-03-26 20:49:38 +01:00
dictDefaultMessage : dropzone . getAttribute ( 'data-default-message' ) ,
dictInvalidFileType : dropzone . getAttribute ( 'data-invalid-input-type' ) ,
dictFileTooBig : dropzone . getAttribute ( 'data-file-too-big' ) ,
dictRemoveFile : dropzone . getAttribute ( 'data-remove-file' ) ,
2023-04-03 12:06:57 +02:00
timeout : 0 ,
thumbnailMethod : 'contain' ,
thumbnailWidth : 480 ,
thumbnailHeight : 480 ,
init ( ) {
this . on ( 'success' , ( file , data ) => {
file . uuid = data . uuid ;
fileUuidDict [ file . uuid ] = { submitted : false } ;
2024-03-26 20:49:38 +01:00
const input = document . createElement ( 'input' ) ;
input . id = data . uuid ;
input . name = 'files' ;
input . type = 'hidden' ;
input . value = data . uuid ;
dropzone . querySelector ( '.files' ) . insertAdjacentHTML ( 'beforeend' , input . outerHTML ) ;
2023-04-03 12:06:57 +02:00
} ) ;
2024-03-15 19:02:43 +01:00
this . on ( 'removedfile' , async ( file ) => {
2023-04-20 08:39:44 +02:00
if ( disableRemovedfileEvent ) return ;
2024-03-26 20:49:38 +01:00
document . getElementById ( file . uuid ) ? . remove ( ) ;
if ( dropzone . getAttribute ( 'data-remove-url' ) && ! fileUuidDict [ file . uuid ] . submitted ) {
2024-03-15 19:02:43 +01:00
try {
2024-03-26 20:49:38 +01:00
await POST ( dropzone . getAttribute ( 'data-remove-url' ) , { data : new URLSearchParams ( { file : file . uuid } ) } ) ;
2024-03-15 19:02:43 +01:00
} catch ( error ) {
console . error ( error ) ;
}
2023-04-03 12:06:57 +02:00
}
} ) ;
this . on ( 'submit' , ( ) => {
2024-03-26 20:49:38 +01:00
for ( const fileUuid of Object . keys ( fileUuidDict ) ) {
2023-04-03 12:06:57 +02:00
fileUuidDict [ fileUuid ] . submitted = true ;
2024-03-26 20:49:38 +01:00
}
2023-04-03 12:06:57 +02:00
} ) ;
2024-03-15 19:02:43 +01:00
this . on ( 'reload' , async ( ) => {
try {
2024-03-26 20:49:38 +01:00
const response = await GET ( editContentZone . getAttribute ( 'data-attachment-url' ) ) ;
2024-03-15 19:02:43 +01:00
const data = await response . json ( ) ;
2023-04-20 08:39:44 +02:00
// do not trigger the "removedfile" event, otherwise the attachments would be deleted from server
disableRemovedfileEvent = true ;
2023-04-03 12:06:57 +02:00
dz . removeAllFiles ( true ) ;
2024-03-26 20:49:38 +01:00
dropzone . querySelector ( '.files' ) . innerHTML = '' ;
2023-04-20 08:39:44 +02:00
fileUuidDict = { } ;
disableRemovedfileEvent = false ;
for ( const attachment of data ) {
2024-03-26 20:49:38 +01:00
const imgSrc = ` ${ dropzone . getAttribute ( 'data-link-url' ) } / ${ attachment . uuid } ` ;
2023-04-20 08:39:44 +02:00
dz . emit ( 'addedfile' , attachment ) ;
dz . emit ( 'thumbnail' , attachment , imgSrc ) ;
dz . emit ( 'complete' , attachment ) ;
dz . files . push ( attachment ) ;
fileUuidDict [ attachment . uuid ] = { submitted : true } ;
2024-03-26 20:49:38 +01:00
dropzone . querySelector ( ` img[src=' ${ imgSrc } '] ` ) . style . maxWidth = '100%' ;
const input = document . createElement ( 'input' ) ;
input . id = attachment . uuid ;
input . name = 'files' ;
input . type = 'hidden' ;
input . value = attachment . uuid ;
dropzone . querySelector ( '.files' ) . insertAdjacentHTML ( 'beforeend' , input . outerHTML ) ;
2023-04-20 08:39:44 +02:00
}
2024-03-15 19:02:43 +01:00
} catch ( error ) {
console . error ( error ) ;
}
2023-04-03 12:06:57 +02:00
} ) ;
} ,
} ) ;
dz . emit ( 'reload' ) ;
return dz ;
} ;
const cancelAndReset = ( dz ) => {
2024-03-26 20:49:38 +01:00
showElem ( renderContent ) ;
hideElem ( editContentZone ) ;
2023-04-03 12:06:57 +02:00
if ( dz ) {
2021-11-18 17:45:00 +01:00
dz . emit ( 'reload' ) ;
}
2023-04-03 12:06:57 +02:00
} ;
2024-03-15 19:02:43 +01:00
const saveAndRefresh = async ( dz ) => {
2024-03-26 20:49:38 +01:00
showElem ( renderContent ) ;
hideElem ( editContentZone ) ;
2024-03-15 19:02:43 +01:00
try {
const params = new URLSearchParams ( {
content : comboMarkdownEditor . value ( ) ,
2024-03-26 20:49:38 +01:00
context : editContentZone . getAttribute ( 'data-context' ) ,
2024-03-15 19:02:43 +01:00
} ) ;
for ( const file of dz . files ) params . append ( 'files[]' , file . uuid ) ;
2024-03-26 20:49:38 +01:00
const response = await POST ( editContentZone . getAttribute ( 'data-update-url' ) , { data : params } ) ;
2024-03-15 19:02:43 +01:00
const data = await response . json ( ) ;
2023-04-03 12:06:57 +02:00
if ( ! data . content ) {
2024-03-26 20:49:38 +01:00
renderContent . innerHTML = document . getElementById ( 'no-content' ) . innerHTML ;
rawContent . textContent = '' ;
2023-04-03 12:06:57 +02:00
} else {
2024-03-26 20:49:38 +01:00
renderContent . innerHTML = data . content ;
rawContent . textContent = comboMarkdownEditor . value ( ) ;
const refIssues = renderContent . querySelectorAll ( 'p .ref-issue' ) ;
attachRefIssueContextPopup ( refIssues ) ;
2023-04-03 12:06:57 +02:00
}
2024-03-26 20:49:38 +01:00
const content = segment ;
if ( ! content . querySelector ( '.dropzone-attachments' ) ) {
2023-04-03 12:06:57 +02:00
if ( data . attachments !== '' ) {
2024-03-26 20:49:38 +01:00
content . insertAdjacentHTML ( 'beforeend' , data . attachments ) ;
2023-04-03 12:06:57 +02:00
}
} else if ( data . attachments === '' ) {
2024-03-26 20:49:38 +01:00
content . querySelector ( '.dropzone-attachments' ) . remove ( ) ;
2023-04-03 12:06:57 +02:00
} else {
2024-03-26 20:49:38 +01:00
content . querySelector ( '.dropzone-attachments' ) . outerHTML = data . attachments ;
2023-04-03 12:06:57 +02:00
}
2021-11-18 17:45:00 +01:00
if ( dz ) {
2023-04-03 12:06:57 +02:00
dz . emit ( 'submit' ) ;
2021-11-18 17:45:00 +01:00
dz . emit ( 'reload' ) ;
}
2023-04-03 12:06:57 +02:00
initMarkupContent ( ) ;
initCommentContent ( ) ;
2024-03-15 19:02:43 +01:00
} catch ( error ) {
console . error ( error ) ;
}
2023-04-03 12:06:57 +02:00
} ;
2022-05-20 04:26:04 +02:00
2024-03-26 20:49:38 +01:00
if ( ! editContentZone . innerHTML ) {
editContentZone . innerHTML = document . getElementById ( 'issue-comment-editor-template' ) . innerHTML ;
comboMarkdownEditor = await initComboMarkdownEditor ( editContentZone . querySelector ( '.combo-markdown-editor' ) ) ;
2023-04-03 12:06:57 +02:00
2024-03-26 20:49:38 +01:00
const dropzone = editContentZone . querySelector ( '.dropzone' ) ;
const dz = await setupDropzone ( dropzone ) ;
editContentZone . querySelector ( '.cancel.button' ) . addEventListener ( 'click' , ( e ) => {
2023-04-03 12:06:57 +02:00
e . preventDefault ( ) ;
cancelAndReset ( dz ) ;
} ) ;
2024-03-26 20:49:38 +01:00
editContentZone . querySelector ( '.save.button' ) . addEventListener ( 'click' , ( e ) => {
2023-04-03 12:06:57 +02:00
e . preventDefault ( ) ;
2024-02-21 01:05:17 +01:00
saveAndRefresh ( dz ) ;
2021-11-18 17:45:00 +01:00
} ) ;
2023-04-03 12:06:57 +02:00
} else {
2024-03-26 20:49:38 +01:00
comboMarkdownEditor = getComboMarkdownEditor ( editContentZone . querySelector ( '.combo-markdown-editor' ) ) ;
2021-11-18 17:45:00 +01:00
}
// Show write/preview tab and copy raw content as needed
2024-03-26 20:49:38 +01:00
showElem ( editContentZone ) ;
hideElem ( renderContent ) ;
2023-04-03 12:06:57 +02:00
if ( ! comboMarkdownEditor . value ( ) ) {
2024-03-26 20:49:38 +01:00
comboMarkdownEditor . value ( rawContent . textContent ) ;
2021-11-18 17:45:00 +01:00
}
2023-04-03 12:06:57 +02:00
comboMarkdownEditor . focus ( ) ;
2021-11-18 17:45:00 +01:00
}
2021-11-09 10:27:25 +01:00
export function initRepository ( ) {
2024-03-25 19:37:55 +01:00
if ( ! $ ( '.page-content.repository' ) . length ) return ;
2021-10-16 19:28:04 +02:00
2023-05-03 23:58:59 +02:00
initRepoBranchTagSelector ( '.js-branch-tag-selector' ) ;
2021-10-16 19:28:04 +02:00
// Options
if ( $ ( '.repository.settings.options' ) . length > 0 ) {
// Enable or select internal/external wiki system and issue tracker.
$ ( '.enable-system' ) . on ( 'change' , function ( ) {
if ( this . checked ) {
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . data ( 'target' ) ) . removeClass ( 'disabled' ) ;
if ( ! $ ( this ) . data ( 'context' ) ) $ ( $ ( this ) . data ( 'context' ) ) . addClass ( 'disabled' ) ;
2021-10-16 19:28:04 +02:00
} else {
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . data ( 'target' ) ) . addClass ( 'disabled' ) ;
if ( ! $ ( this ) . data ( 'context' ) ) $ ( $ ( this ) . data ( 'context' ) ) . removeClass ( 'disabled' ) ;
2021-10-16 19:28:04 +02:00
}
} ) ;
$ ( '.enable-system-radio' ) . on ( 'change' , function ( ) {
if ( this . value === 'false' ) {
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . data ( 'target' ) ) . addClass ( 'disabled' ) ;
2022-11-22 01:58:55 +01:00
if ( $ ( this ) . data ( 'context' ) !== undefined ) $ ( $ ( this ) . data ( 'context' ) ) . removeClass ( 'disabled' ) ;
2021-10-16 19:28:04 +02:00
} else if ( this . value === 'true' ) {
2022-01-16 12:19:26 +01:00
$ ( $ ( this ) . data ( 'target' ) ) . removeClass ( 'disabled' ) ;
2022-11-22 01:58:55 +01:00
if ( $ ( this ) . data ( 'context' ) !== undefined ) $ ( $ ( this ) . data ( 'context' ) ) . addClass ( 'disabled' ) ;
2021-10-16 19:28:04 +02:00
}
} ) ;
2022-06-10 07:39:53 +02:00
const $trackerIssueStyleRadios = $ ( '.js-tracker-issue-style' ) ;
$trackerIssueStyleRadios . on ( 'change input' , ( ) => {
const checkedVal = $trackerIssueStyleRadios . filter ( ':checked' ) . val ( ) ;
$ ( '#tracker-issue-style-regex-box' ) . toggleClass ( 'disabled' , checkedVal !== 'regexp' ) ;
} ) ;
2021-10-16 19:28:04 +02:00
}
// Labels
initCompLabelEdit ( '.repository.labels' ) ;
// Milestones
if ( $ ( '.repository.new.milestone' ) . length > 0 ) {
$ ( '#clear-date' ) . on ( 'click' , ( ) => {
$ ( '#deadline' ) . val ( '' ) ;
return false ;
} ) ;
}
// Repo Creation
if ( $ ( '.repository.new.repo' ) . length > 0 ) {
$ ( 'input[name="gitignores"], input[name="license"]' ) . on ( 'change' , ( ) => {
const gitignores = $ ( 'input[name="gitignores"]' ) . val ( ) ;
const license = $ ( 'input[name="license"]' ) . val ( ) ;
if ( gitignores || license ) {
2024-03-16 16:08:10 +01:00
document . querySelector ( 'input[name="auto_init"]' ) . checked = true ;
2021-10-16 19:28:04 +02:00
}
} ) ;
}
2021-11-18 17:45:00 +01:00
// Compare or pull request
const $repoDiff = $ ( '.repository.diff' ) ;
if ( $repoDiff . length ) {
initRepoCommonBranchOrTagDropdown ( '.choose.branch .dropdown' ) ;
initRepoCommonFilterSearchDropdown ( '.choose.branch .dropdown' ) ;
}
2022-03-29 05:21:30 +02:00
initRepoCloneLink ( ) ;
2022-11-11 18:02:50 +01:00
initCitationFileCopyContent ( ) ;
2021-11-18 17:45:00 +01:00
initRepoSettingBranches ( ) ;
2021-10-16 19:28:04 +02:00
// Issues
if ( $ ( '.repository.view.issue' ) . length > 0 ) {
2021-11-18 17:45:00 +01:00
initRepoIssueCommentEdit ( ) ;
2021-10-16 19:28:04 +02:00
initRepoIssueBranchSelect ( ) ;
initRepoIssueTitleEdit ( ) ;
initRepoIssueWipToggle ( ) ;
initRepoIssueComments ( ) ;
initRepoDiffConversationNav ( ) ;
initRepoIssueReferenceIssue ( ) ;
initRepoIssueCommentDelete ( ) ;
initRepoIssueDependencyDelete ( ) ;
initRepoIssueCodeCommentCancel ( ) ;
initRepoPullRequestUpdate ( ) ;
2023-05-28 03:34:18 +02:00
initCompReactionSelector ( $ ( document ) ) ;
2022-05-12 15:39:02 +02:00
initRepoPullRequestMergeForm ( ) ;
2023-11-02 15:49:02 +01:00
initRepoPullRequestCommitStatus ( ) ;
2021-10-16 19:28:04 +02:00
}
// Pull request
const $repoComparePull = $ ( '.repository.compare.pull' ) ;
if ( $repoComparePull . length > 0 ) {
// show pull request form
$repoComparePull . find ( 'button.show-form' ) . on ( 'click' , function ( e ) {
e . preventDefault ( ) ;
2023-02-19 05:06:14 +01:00
hideElem ( $ ( this ) . parent ( ) ) ;
2021-11-18 17:45:00 +01:00
const $form = $repoComparePull . find ( '.pullrequest-form' ) ;
2023-02-19 05:06:14 +01:00
showElem ( $form ) ;
2021-10-16 19:28:04 +02:00
} ) ;
}
2022-01-07 02:18:52 +01:00
initUnicodeEscapeButton ( ) ;
2021-10-16 19:28:04 +02:00
}
2021-11-18 17:45:00 +01:00
function initRepoIssueCommentEdit ( ) {
// Edit issue or comment content
$ ( document ) . on ( 'click' , '.edit-content' , onEditContent ) ;
2021-10-16 19:28:04 +02:00
// Quote reply
2023-03-02 20:53:22 +01:00
$ ( document ) . on ( 'click' , '.quote-reply' , async function ( event ) {
event . preventDefault ( ) ;
2021-10-16 19:28:04 +02:00
const target = $ ( this ) . data ( 'target' ) ;
2022-12-09 17:25:32 +01:00
const quote = $ ( ` # ${ target } ` ) . text ( ) . replace ( /\n/g , '\n> ' ) ;
2021-10-16 19:28:04 +02:00
const content = ` > ${ quote } \n \n ` ;
2023-04-03 12:06:57 +02:00
let editor ;
2021-10-16 19:28:04 +02:00
if ( $ ( this ) . hasClass ( 'quote-reply-diff' ) ) {
2023-03-02 20:53:22 +01:00
const $replyBtn = $ ( this ) . closest ( '.comment-code-cloud' ) . find ( 'button.comment-form-reply' ) ;
2023-04-03 12:06:57 +02:00
editor = await handleReply ( $replyBtn ) ;
2021-11-18 17:45:00 +01:00
} else {
// for normal issue/comment page
2023-04-03 12:06:57 +02:00
editor = getComboMarkdownEditor ( $ ( '#comment-form .combo-markdown-editor' ) ) ;
2021-10-16 19:28:04 +02:00
}
2023-04-03 12:06:57 +02:00
if ( editor ) {
if ( editor . value ( ) ) {
editor . value ( ` ${ editor . value ( ) } \n \n ${ content } ` ) ;
2021-10-16 19:28:04 +02:00
} else {
2023-04-03 12:06:57 +02:00
editor . value ( content ) ;
2021-10-16 19:28:04 +02:00
}
2023-04-03 12:06:57 +02:00
editor . focus ( ) ;
editor . moveCursorToEnd ( ) ;
2021-10-16 19:28:04 +02:00
}
} ) ;
}